Description
Add CLI & form interface to your program.
fui alternatives and similar packages
Based on the "TUI" category.
Alternatively, view fui alternatives based on common mentions on social networks and blogs.
WorkOS - The modern identity platform for B2B SaaS
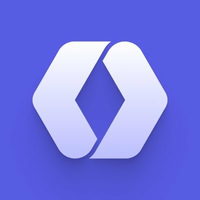
Do you think we are missing an alternative of fui or a related project?
Popular Comparisons
README
fui
Add CLI & form interface to your program.
Basic example
cargo.toml
[dependencies]
fui = "1.0"
Using with clap
(experimental)
extern crate clap;
extern crate fui;
use clap::{App, Arg};
use fui::Fui;
use std::env;
// regular clap code
let app = App::new("some-app").arg(
Arg::with_name("some-switch")
.long("arg-long")
.help("arg-help"),
);
// extra fui code
let mut _arg_vec: Vec<String> = env::args().collect();
if _arg_vec.len() <= 1 {
_arg_vec = Fui::from(&app).get_cli_input();
}
// regular clap code
let matches = app.get_matches_from(_arg_vec);
Using without clap
// Example showing imagined CLI app. with two actions
#[macro_use]
extern crate clap;
extern crate fui;
use fui::{Fui, Value};
use fui::form::FormView;
use fui::fields::Text;
fn hdlr(v: Value) {
println!("user input (from fn) {:?}", v);
}
fn main() {
Fui::new(crate_name!())
.action(
"action1",
"help for action1",
FormView::new().field(Text::new("action1-data").help("help for action1 data")),
|v| {
println!("user input (from closure) {:?}", v);
},
)
.action(
"action2",
"help for action2",
FormView::new().field(Text::new("action2-data").help("help for action2 data")),
hdlr,
)
.version(crate_version!())
.about(crate_description!())
.author(crate_authors!())
.run();
}
This will make the program automatically working in 2 modes:
Ready for parsing CLI arguments, like here:
$ ./app_basic -h app_basic 1.0.0 xliiv <[email protected]> An Example program which has CLI & form interface (TUI) USAGE: app_basic [SUBCOMMAND] FLAGS: -h, --help Prints help information -V, --version Prints version information SUBCOMMANDS: action1 help for action1 action2 help for action2 help Prints this message or the help of the given subcommand(s)
Ready for getting user input from easy and discoverable TUI interface, like image below:
More examples
Screens
Clap support
Implemented features
- switch arguments
- positional arguments
- option arguments
- global arguments
- subcommands (single level)
To be implemented
- conflicts_with
- requires
- validators
- min/max/exact number of values for
- positional args
- options args
- groups
- conditional defaults
- custom delimeter
TODO
- find a solution for long help messages
- ctrl+enter submits (#151)
- handle unwraps
Ideas
.validator(OneOf || Regex::new("v\d+\.\d+\.\d+")).unwrap()
?- support user's history?
- checkboxes: automatic toggle on char(+alt)?
- replace
views::Autocomplete
&views::Multiselect
with a new implementation ofAutocomplete
*Note that all licence references and agreements mentioned in the fui README section above
are relevant to that project's source code only.